Engage Your Students with a Python Programming Lesson Idea
Are you ready to supercharge your computer science and computing classes with an exhilarating Python programming challenge?
Look no further! We have an enticing opportunity that your students will love.
Get ready to embark on a coding adventure perfectly tailored for Key Stage 3 and early GCSE classes.
To kick-start this engaging activity, simply download our free 1-page PDF handout, the Palindrome Challenge. This comprehensive resource includes everything you need to get started.
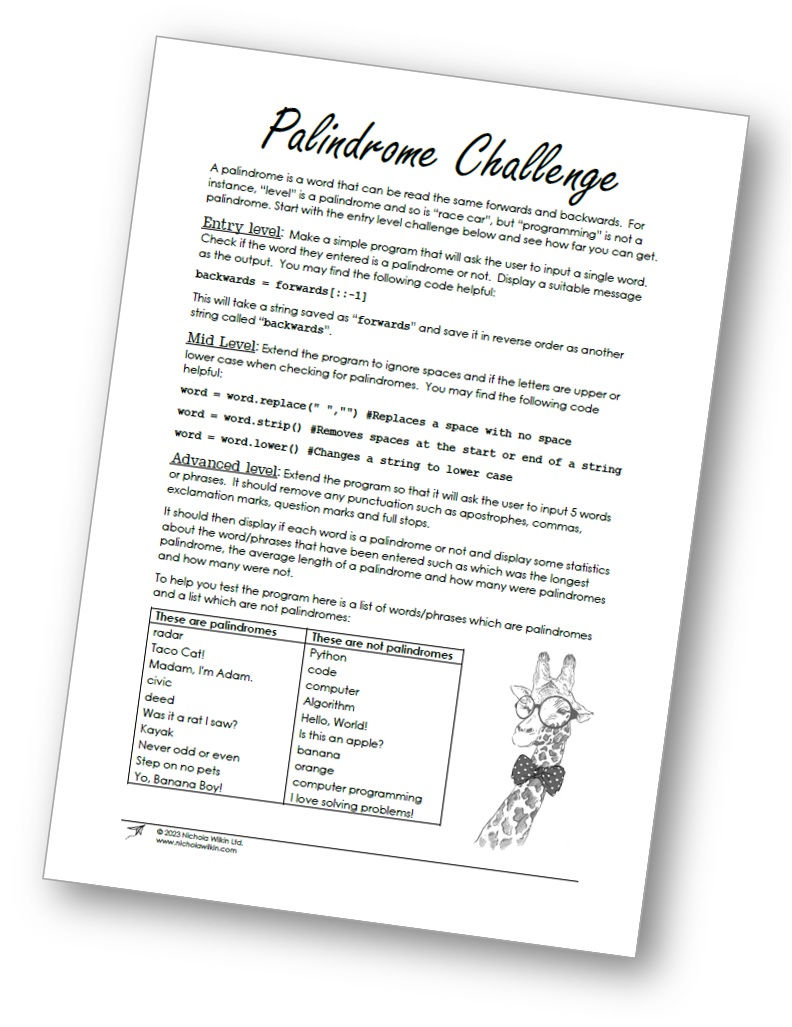
Equipped with the handout, distribute copies to each student and let them dive right into the challenge.
Rest assured, this task aligns seamlessly with your curriculum, reinforcing fundamental Python programming skills. Students will have the chance to practice and demonstrate their abilities in:
Input data
Displaying output with variables
Manipulating strings (case changes, space removal, length calculation, etc.)
Utilizing if statements
Harnessing the power of for loops
Manipulating lists
Once students successfully complete the entry level challenge, they can confidently progress to the mid-level challenge.
Entry Level Python Programming Challenge
In this stage, students are tasked with receiving user input, reversing it (we've even provided the necessary code), and utilising an if statement to present the appropriate output.
Here's the code to jumpstart their progress:
forwards = input("Enter a word: ")
backwards = forwards[::-1]
if forwards == backwards:
print(f"{forwards} is a palindrome")
else:
print(f"{forwards} is not a palindrome")
Once they have completed the entry level challenge they can move onto the mid level challenge.
Mid Level Python Programming Challenge
This stage requires them to tackle character removal and lowercase conversion. We've included the necessary code snippet in the handout. However, students must decide when and how to apply this code while still displaying the original word as initially entered by the user in the final output.
Here's a possible solution for the mid-level challenge:
word = input("Enter a word: ")
forwards = word.replace(" ","")
forwards = forwards.strip()
forwards = forwards.lower()
backwards = forwards[::-1]
if forwards == backwards:
print(f"{word} is a palindrome")
else:
print(f"{word} is not a palindrome")
As students conquer the mid-level challenge, they can take their skills to the next level with the advanced challenge.
Advanced Level Python Programming Challenge
Here, they won't receive any additional code but will be tasked with adapting their existing code to remove punctuation. They will also need to incorporate loops to repeat the code, and provide statistics on the entered words.
Here's one possible solution for the advanced-level Python programming challenge, designed to foster problem-solving skills and reinforce key programming concepts:
words=[]
forwards=[]
backwards = []
for i in range(0,5):
words.append(input("Enter a word: "))
forwards.append(words[i].replace(" ",""))
forwards[i] = forwards[i].replace("'","")
forwards[i] = forwards[i].replace(",","")
forwards[i] = forwards[i].replace("!","")
forwards[i] = forwards[i].replace("?","")
forwards[i] = forwards[i].replace(".","")
forwards[i] = forwards[i].strip()
forwards[i] = forwards[i].lower()
backwards.append(forwards[i][::-1])
print()
count = 0
total_length = 0
max_length = 0
for i in range(0,5):
if forwards[i] == backwards[i]:
print(f"'{words[i]}' is a palindrome")
total_length = total_length + len(forwards[i])
if len(forwards[i]) > max_length:
max_length = len(forwards[i])
count = count +1
else:
print(f"'{words[i]}' is not a palindrome")
print()
print(f"The average length of a palindrom is {total_length/count:.2f} letters")
print(f"The longest palindrom is {max_length} letters")
print(f"{count} words/phrases were palindromes and {5-count} were not.")
Pupils love this Python programming challenge and I’m sure you will too. All you need to do is download the free handout from the link above, print it out and let your pupils work through it for a fun, interactive and highly engaging lesson that helps them embed their programming skills.
Enjoy!
If you would like to discover 3 simple tricks to immediately improve your programming lessons click here
Comments